Python | クラス継承の使い方
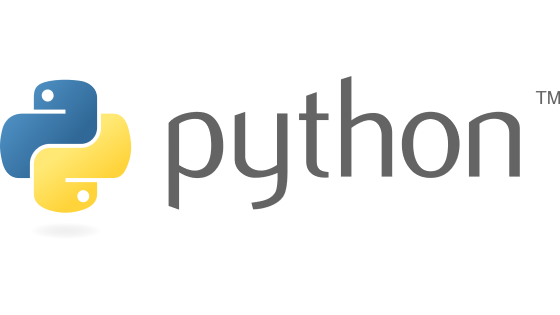
公開日:2021/6/3
Pythonでクラスを用いる際,クラス継承を利用することができる.以下に使い方を記す.
◆実施環境
Python 3.8.8
■クラス継承の基本
クラス継承の特徴は以下になる.
・親クラスのプロパティを子クラスは初期化することができる
・親クラスのインスタンスメソッドを子クラスでも利用できる
・親クラスのインスタンスメソッドを子クラスで新たに定義することができる
親クラスを継承するには,子クラスを作成する際の引数に親クラスのクラス名を以下のように記述する.(例:「class “子クラス名"(親クラス名):」と記述する)
class ABC: # 親クラス
省略
class DEF(ABC): # 親クラスを継承するため,親クラスを引数に記述する
親クラスのプロパティを初期化するには,子クラスでもコンストラクタ(__init__)を用いる.その際,以下のように記述する.
class xxx():
def __init__(self,引数1,引数2,引数3):
super().__init__(引数1,引数2) # 親クラスのインスタンス変数を記載
self.引数3 = 引数3 # 親クラスにないインスタンス変数はここで初期化
以下にクラス継承の構文を作成する
なお,親クラスのインスタンスメソッド(introduction)を子クラスで書き換えることをオーバーライドという.オーバーライドする際には,"super()"を利用する.
# 1の処理
class Bird: # 親クラス
def __init__(self,name,age):
self.name = name
self.age = age
def introduction(self):
print(f'Hi, this is {self.name}')
def intro_age(self):
print(f'I\'m {self.age} years old')
class Crow(Bird): # 子クラス:Birdの機能を継承
def __init__(self,name,age,color):
super().__init__(name,age) # nameとageを初期化.
self.color = color # numberは初期化されていないので,記述する
def intro_color(self):
print(f'my color is {self.color}')
def introduction(self): # オーバーライド(上書きをする)
super().introduction()
print(f'This is crow and color is {self.color}')
Bird1 = Crow('Kataro',10,'black')
Bird1.introduction() # 親クラスと子クラスのprint内容が出力
Bird1.intro_age()
Bird1.intro_color()
■実行結果
# 1の結果
Hi, this is Kataro
This is crow and color is black
I'm 10 years old
my color is black
以上