Python | 特定ファイルから他のファイルの操作方法
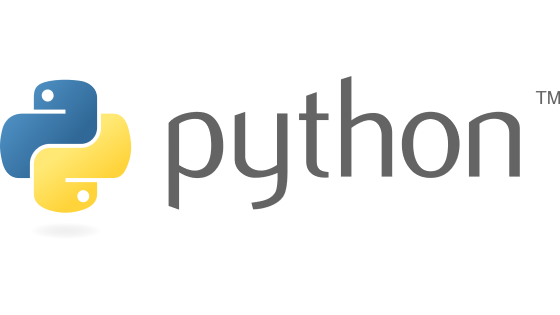
公開日:2021/6/12
Pythonでは特定のファイルから他のファイルを操作することができる.その際に"import"や"as"を利用する.以下にファイルの階層を確認しながら,操作方法を記す.
◆実施環境
Python 3.8.8
■準備
空のフォルダーを作成する.(フォルダー名は"section3″)
“section3″フォルダーをVS Codeから読み込む(File→Open Folder→"section3″フォルダーをダブルクリックをし,"フォルダーの選択"をクリックする).VS Codeは以下の画像になる.
“section3″フォルダー内に,"main.py","sub.py","test"フォルダーを作成する."test"フォルダー内に,"__init__.py","sample1.py","sample2.py"を作成する.
■"sys"の利用
“main.py"にて以下を記載し,ターミナルにて実行をする.
# 1の処理:"sys.path"とは,インタプリターが必要なモジュールを検索する際のディレクトリーのリストが含まれている.そのため,printを利用して,実行しているファイルの場所などを出力する.
# 2の処理:"sys.path.append()"を利用して,ディレクトリーを追加する.
# 1の処理(ファイルの場所などが出力される)
import sys
print(sys.path)
# 2の処理(ディレクトリを追加する)
import sys
print(sys.path)
sys.path.append('c:\\Users\\shiro\\Desktop') # ディレクトリを追加する
print(sys.path)
■実行結果
# 1の結果
['c:\\Users\\shiro\\Desktop\\210517_python development\\section3',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv\\python38.zip',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv\\DLLs',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv\\lib',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv\\lib\\site-packages']
# 2の結果
['c:\\Users\\shiro\\Desktop\\210517_python development\\section3',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv\\python38.zip',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv\\DLLs',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv\\lib',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv',
'C:\\Users\\shiro\\anaconda3\\envs\\djangoenv\\lib\\site-packages',
'c:\\Users\\shiro\\Desktop'] # 追加ディレクトリー
■"main.py"と"sub.py"を利用した出力
“main.py"と"sub.py"は同じフォルダーに格納されている.
(1) “main.py"にて# 1の処理,"sub.py"にて# 1の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#1の結果が出力される.
(2) “main.py"にて# 2の処理,"sub.py"にて# 2の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#2-1の結果が出力される.同条件で,ターミナルにて"sub.py"を実行すると,実行結果の#2-2の結果が出力される.
(3) “main.py"にて# 3の処理,"sub.py"にて# 3の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#3の結果が出力される.
(4) “main.py"にて# 4の処理,"sub.py"にて# 4の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#4の結果が出力される.
(5) “main.py"にて# 5の処理,"sub.py"にて# 5の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#5の結果が出力される.
(6) “main.py"にて# 6の処理,"sub.py"にて# 6の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#6の結果が出力される."*"はsubの中の全ての関数や変数などをimportできるが,明示されないため,エラーの原因になる.
■ main.py
# 1の処理
import sub
# 2の処理
import sub
# 3の処理
import sub
sub.intro_sub()
class_main = sub.ClassSub()
class_main.greeting()
# 4の処理
from sub import intro_sub
intro_sub()
# 5の処理
from sub import intro_sub as insub, ClassSub as clasub
insub()
class_main1 = clasub()
class_main1.greeting()
# 6の処理("*"の利用はエラーの原因となる)
from sub import *
intro_sub()
■ sub.py
# 1の処理
print('Hello from sub.py')
# 2の処理
if __name__ == '__main__':
print('Hello from sub.py')
# 3の処理
def intro_sub():
print('This is Taro from sub.py')
class ClassSub:
def greeting(self):
print('Good morning in ClassSub from sub.py')
# 4の処理
def intro_sub():
print('This is Taro from sub.py')
# 5の処理
def intro_sub():
print('This is Taro from sub.py')
class ClassSub:
def greeting(self):
print('Good morning in ClassSub from sub.py')
# 6の処理
def intro_sub():
print('This is Taro from sub.py')
■実行結果
# 1の結果
Hello from sub.py
# 2-1の結果
なし
# 2-2の結果
Hello from sub.py
# 3の結果
This is Taro from sub.py
Good morning in ClassSub from sub.py
# 4の結果
This is Taro from sub.py
# 5の結果
This is Taro from sub.py
Good morning in ClassSub from sub.py
# 6の結果
This is Taro from sub.py
■"main.py"と"sample1.py","sample2.py"を利用した出力
“main.py"と同じフォルダーに"test"フォルダーが格納されており,"test"フォルダーの中に"sample1.py"と"sample2.py"が格納されている.
(1) “main.py"にて# 1の処理,"sample1.py"にて# 1の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#1の結果が出力される.
(2) “main.py"にて# 1の処理,"sample1.py"にて# 1の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#1の結果が出力される.
(3) “main.py"と"sample1.py","sample2.py"にて# 3の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#3の結果が出力される.
■ main.py
# 1の処理(実行時にフォルダー名が必要)
import test.sample1
test.sample1.greeting_sample1()
# 2の処理(asを利用することで,実行時にフォルダー名を省略)
import test.sample1 as tes_sample1
tes_sample1.greeting_sample1()
# 3の処理(asを利用することで,実行時にフォルダー名と関数名を省略)
from test.sample1 import greeting_sample1 as gre_sample1
from test.sample2 import greeting_sample2 as gre_sample2
gre_sample1()
gre_sample2()
■ sample1.py
# 1の処理
def greeting_sample1():
print('Hello from sample1.py in test folder')
# 2の処理
def greeting_sample1():
print('Hello from sample1.py in test folder')
# 3の処理
def greeting_sample1():
print('Hello from sample1.py in test folder')
■ sample2.py
# 3の処理
def greeting_sample2():
print('Hello from sample2.py in test folder')
■実行結果
# 1の結果
Hello from sample1.py in test folder
# 2の結果
Hello from sample1.py in test folder
# 3の結果
Hello from sample1.py in test folder
Hello from sample2.py in test folder
■"main.py"と"sample1.py","sample2.py","__init__.py"を利用した出力
“main.py"と同じフォルダーに"test"フォルダーが格納されており,"test"フォルダの中に"sample1.py"と"sample2.py","__init__.py"が格納されている.
(1) “main.py"と"sample1.py","__init__.py"にて# 1の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#1の結果が出力される.
(2) “main.py"と"sample2.py","__init__.py"にて# 2の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#2の結果が出力される.
(3) “main.py"と"sample1.py","sample2.py","__init__.py"にて# 3の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#3の結果が出力される.
(4) “main.py"と"sample1.py","sample2.py","__init__.py"にて# 4の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#4の結果が出力される.
(5) “main.py"と"sample1.py","sample2.py","__init__.py"にて# 5の処理を記載し,ターミナルにて"main.py"を実行すると,実行結果の#5の結果が出力される.
■ main.py
# 1の処理(sample1.pyのprintが実行)
from test import *
sample1.greeting_sample1()
# 2の処理(__init__.pyにsample2がimportされていないので,エラーになる)
from test import *
sample2.greeting_sample2()
# 3の処理
from test import sample1_intro
sample1_intro()
from test import sample2_intro
sample2_intro()
# 4の処理(__init__.pyの[]に"sample2_greeting"が含まれていないのでエラーになる)
from test import *
sample1_greeting()
sample2_greeting()
# 5の処理
from test import *
from test import sample2_greeting
sample1_greeting()
sample2_greeting()
■ sample1.py
# 1の処理
def greeting_sample1():
print('Hello from sample1.py in test folder')
# 3の処理
def greeting_sample1():
print('Hello from sample1.py in test folder')
# 4の処理
def greeting_sample1():
print('Hello from sample1.py in test folder')
# 5の処理
def greeting_sample1():
print('Hello from sample1.py in test folder')
■ sample2.py
# 2の処理
def greeting_sample2():
print('Hello from sample2.py in test folder')
# 3の処理
def greeting_sample2():
print('Hello from sample2.py in test folder')
# 4の処理
def greeting_sample2():
print('Hello from sample2.py in test folder')
# 5の処理
def greeting_sample2():
print('Hello from sample2.py in test folder')
■ __init__.py
# 1の処理
from . import sample1
# 2の処理
from . import sample1
# 3の処理
from .sample1 import greeting_sample1 as sample1_intro
from .sample2 import greeting_sample2 as sample2_intro
# 4の処理
from .sample1 import greeting_sample1 as sample1_greeting
from .sample2 import greeting_sample2 as sample2_greeting
__all__ = ['sample1_greeting'] # importする対象を[]に入れると有効になる
# 5の処理
from .sample1 import greeting_sample1 as sample1_greeting
from .sample2 import greeting_sample2 as sample2_greeting
__all__ = ['sample1_greeting'] # importする対象を[]に入れると有効になる
■実行結果
# 1の結果
Hello from sample1.py in test folder
# 2の結果
NameError: name 'sample2' is not defined
# 3の結果
Hello from sample1.py in test folder
Hello from sample2.py in test folder
# 4の結果
Hello from sample1.py in test folder
NameError: name 'sample2_greeting' is not defined
# 5の結果
Hello from sample1.py in test folder
Hello from sample2.py in test folder
以上