Python | Django | テンプレートでの制御文の使い方
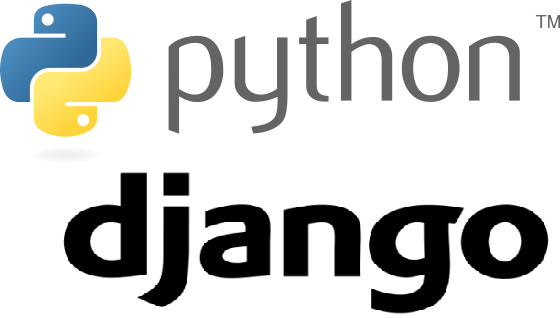
公開日:2021/6/21
Pythonには,DjangoというWebアプリケーションフレームワークがある.フレームワークのため,Djangoを利用するとWebアプリを通常よりも短時間で開発することが可能になる.
前記事にて,Viewの値をテンプレートに渡す方法を記した.前記事での設定をそのまま引き継いだ上で,本記事では,テンプレートでの制御文の使い方を以下2つの構成で記す.
・for文によるループ処理
・if文による制御文
◆実施環境
Python 3.8.8
Django 3.2.3
■for文によるループ処理
“tmp_app/views.py"に新たにリスト"drink_list"を追加する.以下のように編集する.
from django.shortcuts import render
def index(request):
return render(request,'index.html',context={
'greeting_mo':'Good Morning',
'greeting_af':'Good Afternoon',
'greeting_ev':'Good Evening',
'drink_list':['water','coffee','tea','milk'], # 追記箇所
})
“tmp_project/templates/index.html"の9~11行目に"for文"を追記する.index.htmlは以下のようになる.
<html>
<head>
<title>This is how to use templates of tmp_project</title>
</head>
<body>
<h1>Hello World in templates of tmp_project.</h1>
<h1>Which drink is most favorite?</h1> # 以下12行目まで追記箇所
<ul>
{% for drink in drink_list %}
<li>{{ drink }}</li>
{% endfor %}
</ul>
</body>
</html>
“tmp_project/urls.py”は前回編集したので変更することはないが,ブラウザを開く際に”template_app/”が必要なので,現在のコードを以下に記す.
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('template_app/', include('tmp_app.urls')),
]
ターミナルを開き,"conda activate 仮想環境名"を実行し,仮想環境に移行する.”tmp_project”のディレクトリで”python manage.py runserver”を実行すると,以下が出力される.
System check identified no issues (0 silenced).
June 21, 2021 - 14:04:16
Django version 3.2.3, using settings
'tmp_project.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
“http://127.0.0.1:8000/"をクリックし,ブラウザを開くと以下のように表示される.
ブラウザのURLに”http://127.0.0.1:8000/template_app/”を記入し,ブラウザを開くと,"drink_list"の値である"water, coffee, tea, milk"が以下のように出力される.
■if文による制御文
上記にてfor文が記載された"tmp_project/templates/index.html"の14~20行目にif文を追記する."drink_list"に"orange juice"がないので,"My favorite drink is milk!"と出力されるはずである.なお,index.htmlは以下のようになる.
<html>
<head>
<title>This is how to use templates of tmp_project</title>
</head>
<body>
<h1>Hello World in templates of tmp_project.</h1>
<h1>Which drink is most favorite?</h1>
<ul>
{% for drink in drink_list %}
<li>{{ drink }}</li>
{% endfor %}
</ul>
<h1>Answer</h1> # 以下が追記箇所
{% if 'orange juice' in drink_list %}
<h1>My favorite drink is orange juice!</h1>
{% elif 'milk' in drink_list %}
<h1>My favorite drink is milk!</h1>
{% else %}
<h1>My favorite drink is nothing!</h1>
{% endif %}
</body>
</html>
”http://127.0.0.1:8000/template_app/”が記載されたブラウザを更新すると,以下のように出力される."drink_list"に"orange juice"がないので,"My favorite drink is milk!"が出力された.
以上