Python | Django | テンプレートにおけるカスタムフィルタの使い方
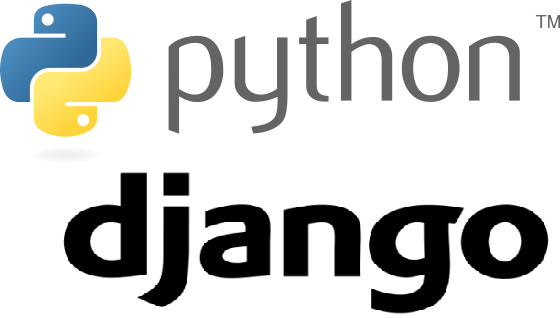
公開日:2021/6/23
Pythonには,DjangoというWebアプリケーションフレームワークがある.フレームワークのため,Djangoを利用するとWebアプリを通常よりも短時間で開発することが可能になる.
前記事にて,テンプレートのおけるタグ・リファレンスの使い方を記した.前記事での設定をそのまま引き継いだ上で,本記事では,テンプレートのおけるカスタムフィルタを説明する.
◆実施環境
Python 3.8.8
Django 3.2.3
■カスタムフィルタの使い方
“tmp_app"フォルダに"templatetags"フォルダを作成する.その中に,"__init__.py"と"cutom_filter.py"を作成する.
“cutom_filter.py"に"from django import template"と"register = template.Library()"を記述し,カスタムフィルタを記述する.この場合のカスタムフィルタは"age_check"となり,変数"age"(年齢)によって,以下のように出力されるフィルタを作成した.
4歳以下:’childhood(幼年期)’
14歳以下:’boyhood(少年期)’
29歳以下:’period of youth(青年期)’
44歳以下:’stage of maturity(壮年期)’
64歳以下:’midlife stage(中年期)’
それ以外:’later in life(高齢期)’
上記記述後の"cutom_filter.py"の内容は以下のようになる.
from django import template
register = template.Library()
@register.filter(name='age_check')
def moving_age_check(age):
if age <= 4:
return 'childhood(幼年期)'
elif age <= 14:
return 'boyhood(少年期)'
elif age <= 29:
return 'period of youth(青年期)'
elif age <= 44:
return 'stage of maturity(壮年期)'
elif age <= 64:
return 'midlife stage(中年期)'
else:
return 'later in life(高齢期)'
“tmp_app/views.py"の"index"関数に以下のように「’age’:25」(年齢:25)を追記する.
def index(request):
return render(request,'index.html',context={
'greeting_mo':'Good Morning',
'greeting_af':'Good Afternoon',
'greeting_ev':'Good Evening',
'drink_list':['water','coffee','tea','milk'],
'age': 25 # 追記箇所
})
“tmp_project/templates/index.html"に"{{ age }}"を記述する."index.html"の内容は以下のようになる.
<html>
<head>
<title>This is how to use templates of tmp_project</title>
</head>
<body>
<h1>Hello World in templates of tmp_project.</h1>
<h1>Which drink is most favorite?</h1>
<ul>
{% for drink in drink_list %}
<li>{{ drink }}</li>
{% endfor %}
</ul>
<h1>Answer</h1>
{% if 'orange juice' in drink_list %}
<h1>My favorite drink is orange juice!</h1>
{% elif 'milk' in drink_list %}
<h1>My favorite drink is milk!</h1>
{% else %}
<h1>My favorite drink is nothing!</h1>
{% endif %}
</body>
<h1>{{ age }}</h1> # 追記箇所
</html>
ターミナルを開き,”conda activate 仮想環境名”を実行し,仮想環境に移行する.”tmp_project”のディレクトリで”python manage.py runserver”を実行すると,以下が出力される.
System check identified no issues (0 silenced).
June 23, 2021 - 18:40:45
Django version 3.2.3, using settings 'tmp_project.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
“http://127.0.0.1:8000/”をクリックし,ブラウザを開くと以下のように表示される.
ブラウザのURLに”http://127.0.0.1:8000/template_app”を入力し,ブラウザを開くと,”index.html”に記述した内容が以下のように出力される.
上記で"tmp_tag"フォルダに"custom_filter.py"を作成したので,"tmp_project/templates/index.html"の一番上に"{{% load custom_filter %}"を記述する.また,"{{ age }}"にカスタムフィルタである"age_check"を追記し,"{{ age | age_check }}"と変更する."index.html"全体は以下のようになる.
{% load custom_filter %} # 追記箇所
<html>
<head>
<title>This is how to use templates of tmp_project</title>
</head>
<body>
<h1>Hello World in templates of tmp_project.</h1>
<h1>Which drink is most favorite?</h1>
<ul>
{% for drink in drink_list %}
<li>{{ drink }}</li>
{% endfor %}
</ul>
<h1>Answer</h1>
{% if 'orange juice' in drink_list %}
<h1>My favorite drink is orange juice!</h1>
{% elif 'milk' in drink_list %}
<h1>My favorite drink is milk!</h1>
{% else %}
<h1>My favorite drink is nothing!</h1>
{% endif %}
</body>
<h1>{{ age | age_check }}</h1> # 変更箇所
</html>
ブラウザのURLに”http://127.0.0.1:8000/template_app”を入力し,ブラウザを開くと,”index.html”に記述した内容が以下のように出力される."age:25″だったため,"period of youth(青年期)"が出力された.
以上