Python | クラス変数とインスタンス変数の使い方
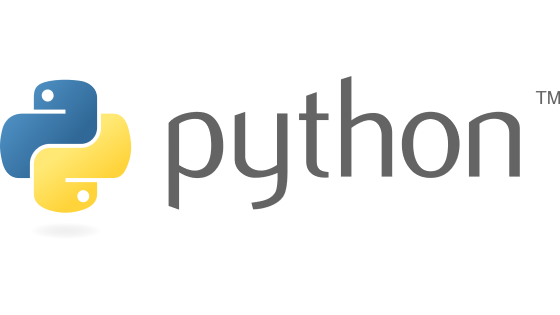
公開日:2021/6/1
Pythonでクラスを用いる際,クラス変数とインスタンス変数がある.以下にクラス変数とインスタンス変数について説明する.
◆実施環境
Python 3.8.8
■クラス変数とインスタンス変数を用いた構文作成
クラスを用いる際のクラス変数とインスタンス変数を用いた構文を以下に作成する.なお,インスタンス変数はインスタンスメソッドの中に記載をする.クラス変数はクラスの次行に記載をする.クラス変数はすべてのインスタンス変数間で利用することができる.クラス変数を書き換えたりすることは構文のミスにつながるので,注意が必要である.
# 1の処理
class Chocolate():
msg = 'Good morning' # クラス変数
def __init__(self,brand,year):
self.brand = brand # インスタンス変数
self.year = year
def greeting(self):
self.msg2 = 'Goodbye' # インスタンス変数
def introduction(self): # インスタンスメソッド
print(f'{self.msg},{self.brand}は{self.year}年に発売開始されました')
Choco1 = Chocolate('Fran',1999)
Choco2 = Chocolate('GABA',2005)
print(Choco1.brand) # 'Fran'が出力
print(Choco2.brand) # 'GABA'が出力
print(Choco1.year) # '1999'が出力
print(Choco2.year) # '2005'が出力
Choco1.greeting()
print(Choco1.msg2) # 'Goodbye'が出力
Choco2.greeting()
print(Choco2.msg2) # 'Goodbye'が出力
Choco1.introduction() # 関数'introduction'の内容(13行目)が出力
Choco2.introduction() # 関数'introduction'の内容(13行目)が出力
print(Chocolate.msg) # クラス変数(msg)の呼び出し方1
print(Choco1.__class__.msg) # クラス変数(msg)の呼び出し方2
Choco3 = Chocolate('Fran',1999) # インスタンスの生成
Choco3.greeting()
Choco3.introduction() # 関数'introduction'の内容(13行目)が出力
Choco1.__class__.msg = 'Good evening' # クラス変数の書き換え.クラス変数は書き換えないほうがよい.
Choco3.introduction() # 関数'introduction'の'msg'が書き換わった内容(13行目)で出力
■実行結果
# 1の結果
Fran
GABA
1999
2005
Goodbye
Goodbye
Good morning,Franは1999年に発売開始されました
Good morning,GABAは2005年に発売開始されました
Good morning
Good morning
Good morning,Franは1999年に発売開始されました
Good evening,Franは1999年に発売開始されました
以上